In one of my larger Matlab applications – Integration-lab Debriefing System (IDS, which shall be described in a future dedicated article) – I wanted to present a panel-level checkbox that applies to the entire panel contents. In my particular case, the IDS uipanel contained a Java table (another future topic) with multiple selectable rows, and I needed a global checkbox that selects all (or none) of them at once:
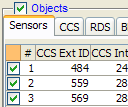
Panel-level ("Objects") checkbox
One way to do this is to calculate the checkbox’s desired position relative to the uipanel and place a regular checkbox-style uicontrol there. The checkbox can even be made a child of the uipanel with ‘normalized’ units, thereby moving and resizing it together with its uipanel parent when the later is moved or resized.
But there’s a much simpler method that want to share. It relies on the undocumented fact that the uipanel‘s title label is a simple hidden uicontrol child of the uipanel handle. This uicontrol handle can be found and simply transformed from a ‘style’='text’ control into a ‘style’='checkbox’ control, as follows:
% Prepare the panel hPanel = uipanel('position',[0.2,0.2,0.4,0.4], 'title','Objects'); % Get the title label's handle warning off MATLAB:Uipanel:HiddenImplementation % turn off warning hTitle = setdiff(findall(hPanel),hPanel); % retrieve title handle hTitle = get(hPanel,'TitleHandle'); % alternative, uses hidden prop % Modify the uicontrol style; add 20 pixel space for the checkbox newPos = get(hTitle,'position') + [0,0,20,0]; % in pixels set(hTitle, 'style','checkbox', 'value',1, 'pos',newPos);
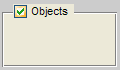
Panel-level checkbox
Note that we can retrieve the title handle using either the uipanel‘s hidden property TitleHandle, or by scanning the panel’s children using findall. I prefer the TitleHandle approach because it does not require modification (minor as it might be) when the panel already contains other children.
The down-side is that since TitleHandle is an undocumented hidden property, it may change its name, its behavior or even be removed in some future Matlab release. In fact, there’s even a Matlab warning about this, unless you turn it off using:
warning off MATLAB:Uipanel:HiddenImplementation
We can use this approach for more complex options panels, as the following example illustrates. Here, we set radio-button controls rather than a checkbox control, and also modify the title color to blue:
% Main panel and basic alternative control hPanelMain = uipanel('pos',[.1,.1,.8,.8], 'Title','Main', 'units','norm'); hAlt1 = uicontrol('parent',hPanelMain, 'units','norm', 'pos',[.1,.8,.5,.1], 'style','radio', 'string','Alternative #1'); % Alternative options panel #2 hAlt2 = uipanel('parent',hPanelMain, 'units','norm', 'pos',[.07,.4,.5,.35], 'title','Alternative #2'); hAlt2Title = get(hAlt2, 'TitleHandle'); newPos = get(hAlt2Title,'position') + [0,0,20,0]; % in pixels set(hAlt2Title, 'style','radio', 'pos',newPos); hAlt2a = uicontrol('parent',hAlt2, 'units','norm', 'pos',[.2,.6,.7,.3], 'style','checkbox', 'string','Option 1'); hAlt2b = uicontrol('parent',hAlt2, 'units','norm', 'pos',[.2,.2,.7,.3], 'style','checkbox', 'string','Option 2'); % Alternative options panel #3 hAlt3 = uipanel('parent',hPanelMain, 'units','norm', 'pos',[.07,.05,.5,.3], 'title','Alternative #3'); hAlt3Title = get(hAlt3, 'TitleHandle'); newPos = get(hAlt3Title,'position') + [0,0,20,0]; % in pixels set(hAlt3Title, 'style','radio', 'pos',newPos, 'ForegroundColor','blue'); hAlt3a = uicontrol('parent',hAlt3, 'units','norm', 'pos',[.2,.5,.7,.3], 'style','popup', 'string',{'Option 3a','Option 3b','Option 3c'});
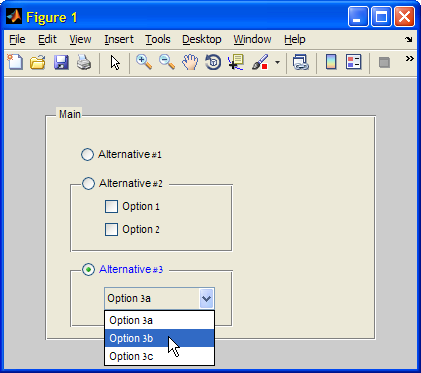
Advanced panel-level controls
Note that since the hAlt2Title and hAlt3Title radio-buttons are children of their respective uipanel parents, we cannot use a simple uibuttongroup to group them in a mutual-exclusion group. Instead, we must use a dedicated callback function. This is actually quite easy:
% Set the callback for all relevant radio-buttons hButtonGroup = [hAlt1, hAlt2Title, hAlt3Title]; set(hButtonGroup, 'Callback', {@SelectionCb, hButtonGroup}); % This is the callback function that manages mutual exclusion function SelectionCb(hSrc,hEvent,hButtonGroup) otherButtons = setdiff(hButtonGroup,hSrc); set(otherButtons,'value',0); set(hSrc,'value',1); % needed to prevent de-selection end
Related posts:
- Images in Matlab uicontrols & labels Images can be added to Matlab controls and labels in a variety of manners, documented and undocumented. ...
- Rich-contents log panel Matlab listboxes and editboxes can be used to display rich-contents HTML-formatted strings, which is ideal for log panels. ...
- GUI integrated HTML panel Simple HTML can be presented in a Java component integrated in Matlab GUI, without requiring the heavy browser control....
- Transparent uipanels Matlab uipanels can be made transparent, for very useful effects. ...