Every now and then, a user asks whether it is possible to make an entire Matlab window transparent (example). This could be used, for example, for window fade-in/fade-out effects. The short answer is that there is no supported way of doing this with pure documented Matlab, but it is trivially easy to achieve using just a bit of Java magic powder (surprise, surprise).
Matlab figure window transparency
Following an idea I got from Malcolm Lidierth’s MUtilities submission on the Matlab File Exchange, the solution for setting Matlab figure window transparency is quite simple: Get the figure’s underlying Java window reference handle, as in last week’s article. Then use Java’s setWindowOpacity method to set the window’s transparency value. Actually, setWindowOpacity sets the opacity level, rather than transparency, but they are obviously complementary and I personally find “transparency” to be more easily understandable.
By default, windows are created with an opacity of 1.0 (= not transparent). They can be set to any floating-point value between 0.0-1.0, where an opacity of 0.0 means full transparency, and any value in between means partial transparency (i.e., translucency):
jFigPeer = get(handle(gcf),'JavaFrame'); jWindow = jFigPeer.fFigureClient.getWindow; com.sun.awt.AWTUtilities.setWindowOpacity(jWindow,0.7)
Similarly, you can set the entire Matlab Desktop’s transparency/opacity value:
jDesktop = com.mathworks.mde.desk.MLDesktop.getInstance.getMainFrame; com.sun.awt.AWTUtilities.setWindowOpacity(jDesktop, 0.8);
Note that the com.sun.awt.AWTUtilities
class also enables other GUI effects that would make a Matlab GUI developer’s mouth to start drooling: shaped windows, per-pixel transparency values, mirroring/reflection, window shadows, gradients etc. Perhaps I’ll explore their adaptation for Matlab figures someday.
Fade-in / fade-out
Window fade-in/fade-out effects can easily be achieved using transparency: Simply invoke the setWindowOpacity method several times, with progressively higher or lower values. This is easily done in a simple blocking loop. For example, to fade-out a window:
for stepIdx = 1 : 5 newAlpha = 1.0 - 0.2*stepIdx; com.sun.awt.AWTUtilities.setWindowOpacity(jWindow,newAlpha); jWindow.repaint; pause(0.2); % seconds end
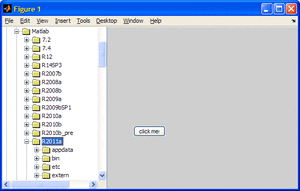
Gradual window fade-out
A more general example dynamically computes the opacity step size/duration and also enables non-blocking fade effects using an asynchronous timer:
% Compute the required opacity-setting steps fadeDuration = 1.5; % seconds oldAlpha = com.sun.awt.AWTUtilities.getWindowOpacity(jWindow); newAlpha = 0.0; deltaAlpha = newAlpha - oldAlpha; maxStepAlpha = 0.03; steps = fix(abs(deltaAlpha) / maxStepAlpha) + 1; stepAlpha = deltaAlpha / steps; stepDuration = fadeDuration / (steps-1); % If blocking, do the fade effect immediately if blockingFlag || steps==1 for stepIdx = 1 : steps newAlpha = oldAlpha + stepAlpha*stepIdx; com.sun.awt.AWTUtilities.setWindowOpacity(jWindow,newAlpha); jWindow.repaint; if stepIdx < steps, pause(stepDuration); end end else % non-blocking: fade in/out asynchronously using a dedicated timer start(timer('ExecutionMode','fixedRate', 'Period',0.1, 'TasksToExecute',steps, ... 'TimerFcn', {@timerFcn,jWindow,oldAlpha,stepAlpha})); end % Timer function for non-blocking fade-in/fade-out effect function timerFcn(hTimer,eventData,jFrame,currentAlpha,stepAlpha) %#ok<INUSL> eventData stepIdx = hTimer.TasksExecuted; newAlpha = currentAlpha + stepAlpha*stepIdx; com.sun.awt.AWTUtilities.setWindowOpacity(jFrame,newAlpha); jFrame.repaint; if stepIdx == hTimer.TasksToExecute stop(hTimer); delete(hTimer); end end % timerFcn
Of course, you can also fade-in/out to intermediate values such as 0.3 or 0.8. If you fade-out completely (i.e., to a value of 0.0), it might be a good idea to actually close the figure window once it gets the totally-transparent value of 0.0.
I’ve prepared a Matlab utility that contains all these options, including optional blocking/non-blocking fade effects, in my setFigTransparency utility, which is available for download on the Matlab File Exchange. You may also wish to use Malcolm Lidierth’s MUtilities, which also has similar functionalities (plus some other goodies).
Limitations
Setting a figure window’s transparency requires using Java Run-time Engine (JRE) 1.6.0_10 (also called “Java 6 update 10″) or higher. This means that it’s supported on Matlab release 7.9 (R2009b) and higher by default, and on earlier releases using a JRE retrofit.
If you are using an earlier Matlab release, consider a retrofit of JRE 1.6.0_10 or any later version (e.g., the latest available version today is 1.6 update 24). The JRE can be downloaded from here, and you can configure Matlab to use it according to the instructions here. As noted, Matlab R2009b (7.9) and onward, at least on Microsoft Windows, pre-bundle a JRE version that does support transparency/opacity and so do not require a retrofit.
You can check your current Java version in Matlab as follows:
>> version -java ans = Java 1.6.0_17-b04 with Sun Microsystems Inc. Java HotSpot(TM) Client VM mixed mode
Unfortunately, Matlab plot axes cannot be made transparent. If you have any axes in your GUI, the axes area will simply appear as a shaded axes, whose intensity depends on the selected alpha (transparency) value; the contents beneath the window will not be merged in the axes area as it is in the non-axes areas.
Finally, note that com.sun.awt.AWTUtilities
is itself an undocumented Java class. It is bundled with the standard Java release since 2008 (1.6.0_10), and yet is not part of the official release because its API has not yet settled. In fact, in the upcoming Java 7 release, which is expected in a few months, and which I expect to be available in Matlab sometime in 2012, the set of transparency/opacity methods have migrated to the fully-documented java.awt.Window
class.
Blurred figure window
So here’s a riddle for you: using figure window transparency, can you guess how to make a Matlab figure appear blurred for disabled figures (see the screenshot there)? There are several possible ways to do this – can you find the simplest? The first one to post a comment with a correct answer gets a smiley… My answer will appear in next week’s article.
Upgraded website
Also, did you notice my new website design? It’s supposed to be much more readable (yes – also on Android…). It now also runs on a multi-server cloud, which means more stability and faster response times. Do you like the new design? hate it? I would love to hear your feedback via comment or email.
Related posts:
- Blurred Matlab figure window Matlab figure windows can be blurred using a semi-transparent overlaid window - this article explains how...
- Minimize/maximize figure window Matlab figure windows can easily be maximized, minimized and restored using a bit of undocumented magic powder...
- Enable/disable entire figure window Disabling/enabling an entire figure window is impossible with pure Matlab, but is very simple using the underlying Java. This article explains how....
- Detecting window focus events Matlab does not have any documented method to detect window focus events (gain/loss). This article describes an undocumented way to detect such events....